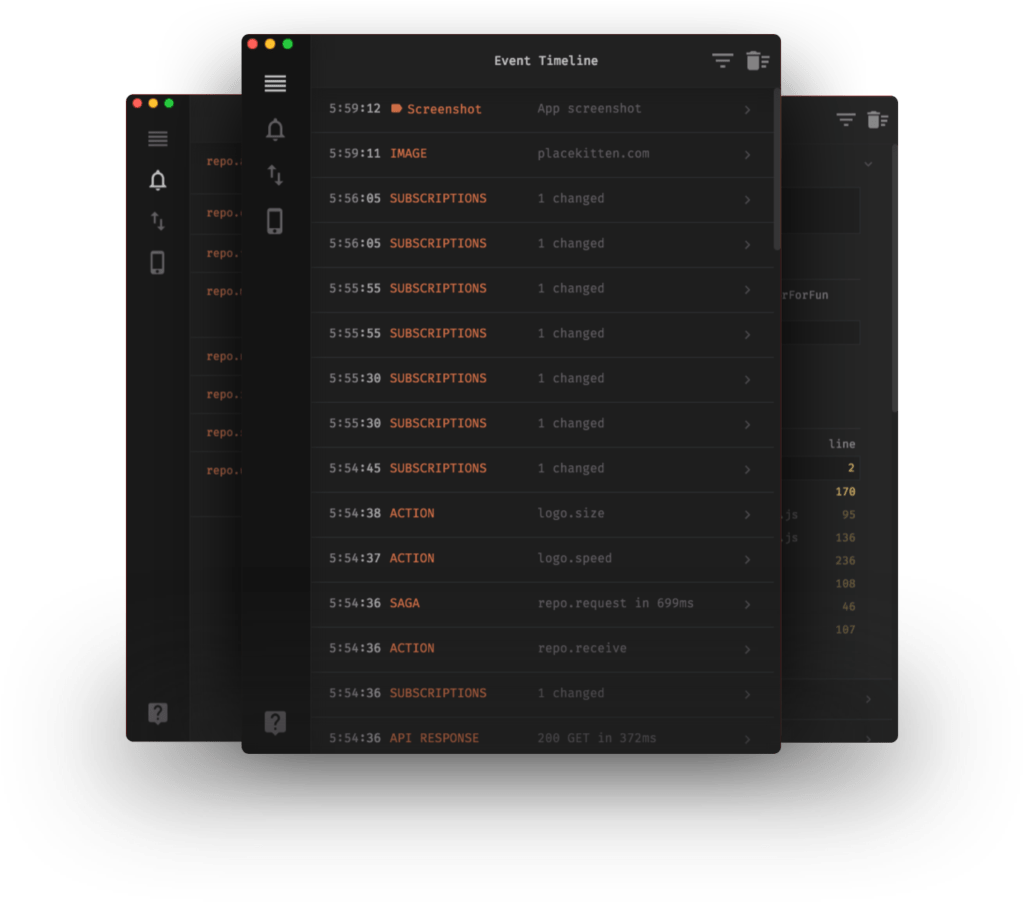
Inspecting apps is now as easy as it was always meant to be. Effortlessly inspect React JS and React Native mobile apps with Reactotron, a free desktop application.
Reactotron can help you debug better by providing a lot of options. For starter, you can debug redux actions, your redux state and even your async storage in React native.
- Let’s download the desktop app to start. You can download for Linux, Windows, and Mac.
- Unzip & run.
- Setting it up is pretty simple, open your app root in terminal.
- Run below command to install Reacotron:
npm i --save-dev reactotron-react-native
- Create a config file for reactotron in your app code with the following code. You can customize the options by referring to documentation.
import Reactotron from 'reactotron-react-native'
Reactotron
.setAsyncStorageHandler(AsyncStorage) // AsyncStorage would either come from `react-native` or `@react-native-community/async-storage` depending on where you get it from
.configure({
name: "React Native Demo"
})
.useReactNative({
asyncStorage: false, // there are more options to the async storage.
networking: { // optionally, you can turn it off with false.
ignoreUrls: /symbolicate/
},
editor: false, // there are more options to editor
errors: { veto: (stackFrame) => false }, // or turn it off with false
overlay: false, // just turning off overlay
})
.connect();
- Configure redux in Reactotron by running the below command:
npm install --save-dev reactotron-redux
- Import this module in reactotron config file like below:
const reactotron = Reactotron
.configure({ name: 'React Native Demo' })
.use(reactotronRedux()) // <- here i am!
.connect()
/* Then in your createStore file, add this */
const store = createStore(rootReducer, Reactotron.createEnhancer())
Now you can see state tab in your Reactotron app where you can see your state and store snapshots, subscribe to changes and even dispatch some actions again directly from here.
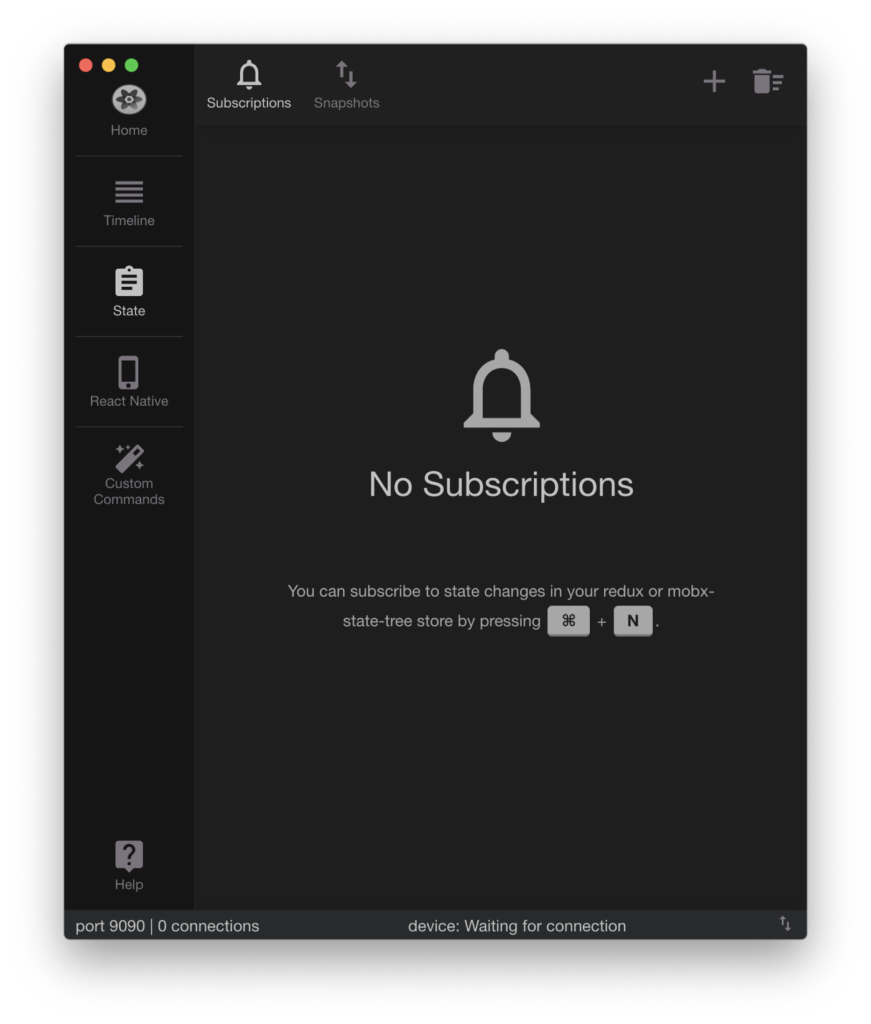
Similar to React Native, Reactotron can be configured for ReactJS Web Apps as well. You can refer ReactJS Docs for Reactotron.