Hello World: The First Step in Programming
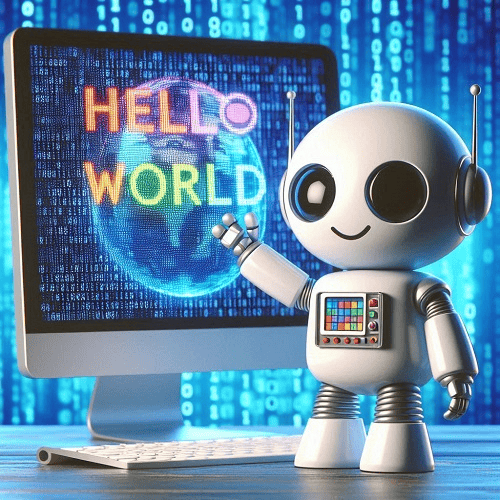
“Hello, World!” is more than just a phrase; it’s a rite of passage for anyone stepping into the world of programming. This simple program is often the first one written by beginners, regardless of the programming language they are learning. It serves as a gentle introduction to the syntax and structure of a new language, providing a sense of accomplishment and a foundation to build upon.
Why “Hello, World!”?
The tradition of using “Hello, World!” dates back to the early days of programming. It was popularized by Brian Kernighan in the 1970s in his book “The C Programming Language.” The simplicity of the program makes it an ideal starting point, allowing beginners to focus on understanding the basic elements of coding without being overwhelmed by complexity.
Writing “Hello, World!” in Different Languages
Let’s explore how to write a “Hello, World!” program in a few popular programming languages:
- Next.js
- Set up your environment:
npx create-next-app@latest hello-world cd hello-world npm run dev
- Edit
pages/index.js
- Set up your environment:
export default function Home() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
- React
- Set up your environment:
npx create-react-app hello-world cd hello-world npm start
- Edit
src/App.js
- Set up your environment:
import React from 'react';
function App() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
export default App;
- React Native
- Set up your environment:
npx react-native init HelloWorld cd HelloWorld npx react-native run-android # or run-ios
- Edit
App.js
- Set up your environment:
import React from 'react';
import { Text, View } from 'react-native';
const App = () => {
return (
<View>
<Text>Hello, World!</Text>
</View>
);
};
export default App;
- PHP
- Set up your environment: Ensure you have a local server like XAMPP or WAMP installed.
- Create a file
index.php
<!DOCTYPE html>
<html>
<head>
<title>Hello, World!</title>
</head>
<body>
<?php
echo 'Hello, World!';
?>
</body>
</html>
- Kotlin
- Set up your environment: Ensure you have Kotlin installed.
- Create a file
HelloWorld.kt
:
fun main() {
println("Hello, World!")
}
- Flutter
- Set up your environment:
flutter create hello_world cd hello_world flutter run
- Edit
lib/main.dart
- Set up your environment:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('Hello, World!'),
),
body: const Center(
child: Text('Hello, World!'),
),
),
);
}
}
- Ruby
puts "Hello, World!"
- C++
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- JavaScript
console.log("Hello, World!");
- Python
print("Hello, World!")
- Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The Significance of “Hello, World!”
Writing a “Hello, World!” program might seem trivial, but it holds significant value for beginners:
- Understanding Syntax: It helps new programmers get familiar with the syntax of a new language.
- Building Confidence: Successfully running the program provides a sense of achievement and boosts confidence.
- Learning Environment Setup: It ensures that the development environment is correctly set up and ready for more complex projects.
Conclusion
“Hello, World!” is more than just a simple program; it’s a symbol of the beginning of a journey into the fascinating world of programming. Whether you’re learning Python, JavaScript, Java, C++, or Ruby, this humble program is your first step towards mastering a new language. So, go ahead, write your “Hello, World!” program, and take your first step into the exciting world of coding!
Latest Highlights
- How To Build Stunning Web Apps: Tools, Steps and Examples
- Llama API for Developers
- Firebase Studio: The full stack AI App Development workspace
- Meta’s Llama 4: Revolutionising AI Code Generation Tools with Massive Context Windows
- Gemini 2.5 Pro: The Future of AI Reasoning
- GPT-4o Just Got an Insane Upgrade: Native Image Generation is Here!
Categories
Start Your Coding Journey with Hello World!
Ready to take your first step into the world of programming?
Write your first "Hello, World!" program and experience the joy of coding. No matter the language—Python, JavaScript, Java, C++, or Ruby—it all begins with this simple yet iconic phrase.
Ready to code?
We can help you. Your programming adventure starts now!